Consider the below points on component rendering
- When a web page loads, all component renders - This is called initial render
- A component re-renders whenever its state or prop changes - This is called subsequent render
- If a component renders, then all of its child component also renders
Notice the last point: the children component re-renders unnecessarily, even if no change happens in it. Let me demonstrate this below.
Before using React memo
Let's say there are four components, nested in below order App -> A -> B -> C I update the 'A' component state on button click. Then unnecessarily 'B' and 'C' component also re-renders with 'A'
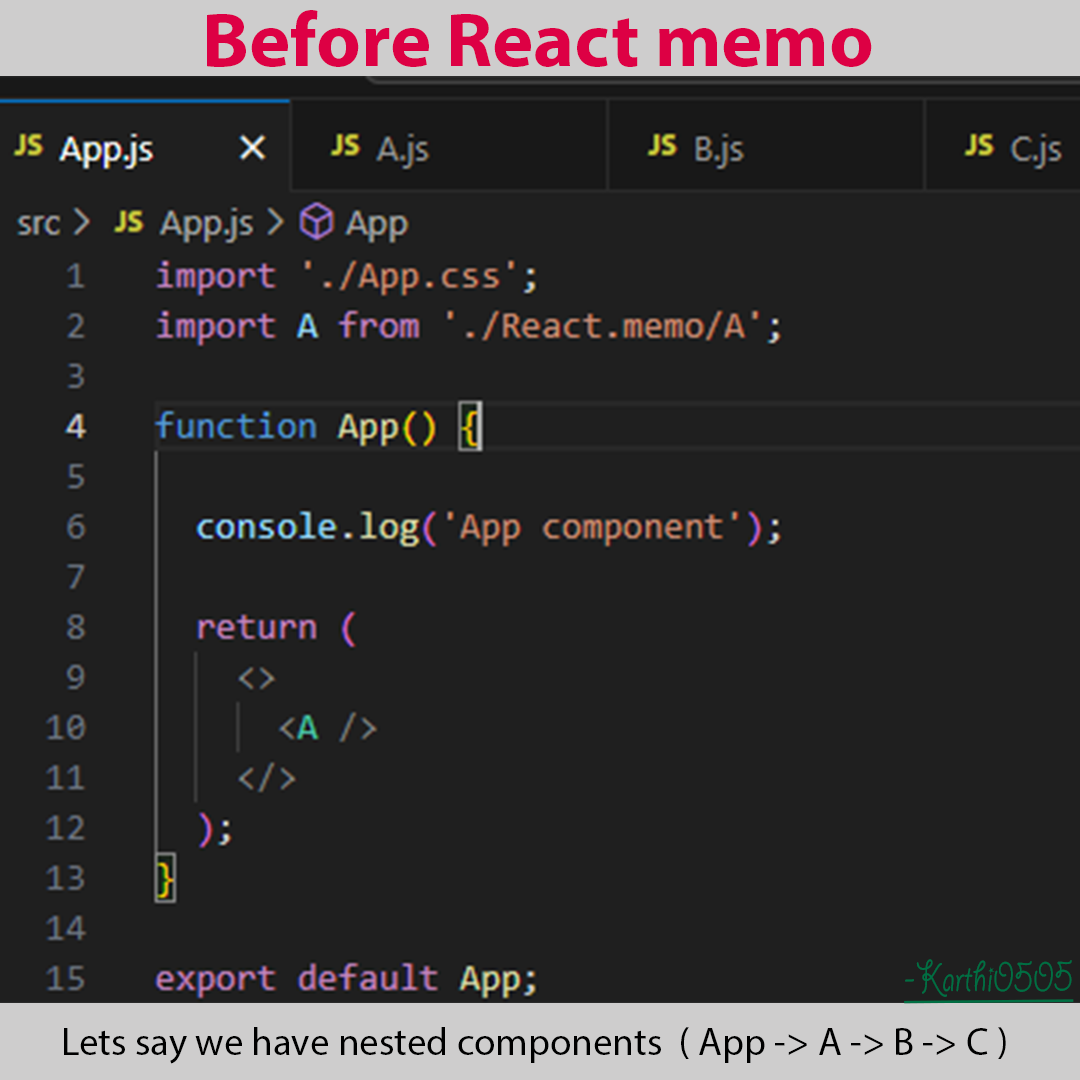
After React memo
React memo prevents unnecessary re-rendering of child component
How memo works
- Memoization: When you use React.memo to wrap a functional component, it creates a new memoized version of that component.
- Props Comparison: When the memoized component is rendered, React.memo compares the current props with the previous props using a shallow equality check.
- Render Decision: If the props have not changed since the last render, React.memo returns the cached result (the memoized component) without re-rendering the wrapped component. This helps to avoid unnecessary rendering.
- Re-render Trigger: If the props have changed, React.memo allows the wrapped component to re-render with the new props, and the result is cached for future comparisons.
Returns
memo returns a new memoized React component.
After React memo - with props passed
Note:
It's important to use it judiciously. Not all components need to be memoized, especially if they are simple or don't render frequently. Always profile and measure performance before and after optimizations to ensure that they are providing the expected benefits.